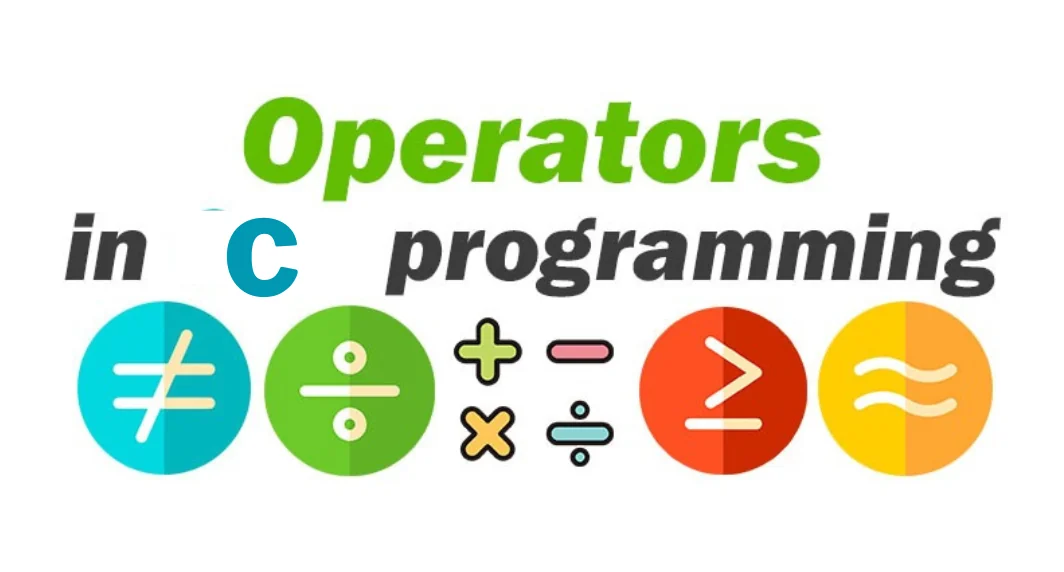
The C programming language utilizes operators as symbols representing precise operations to be executed on one or more operands. C provides a wide range of operators that can perform arithmetic, logical, and bitwise operations and operations on pointers and arrays. Operators are symbols that help in performing functions of mathematical and logical nature.
Use of Operators in C
The operators basically serve as symbols that operate on any value or variable. We use it for performing various operations- such as logical, arithmetic, relational, and many more. A programmer must use various operators for performing certain types of mathematical operations. Thus, the primary purpose of the operators is to perform various logical and mathematical calculations.
The programming languages like C come with some built-in functions that are rich in nature. The use of these operators is vast. These operators act as very powerful and useful features of all the programming languages, and the functionality of these languages is pretty much useless without these. These make it very easy for programmers to write the code very easily and efficiently.
Types of Operators in C
Various types of operators are available in the C language that helps a programmer in performing different types of operations. We can handle different operations in a program using these types of operators:
- Relational Operators
- Arithmetic Operators
- Logical Operators
- Assignment Operators
- Bitwise Operators
- Misc Operators
Even though there are many operators, the execution of these operations happens based on the precedence given to them. Precedence is the order in which the compiler executes the code comprising numerous operators.
Arithmetic Operators
An operator is a special symbol that tells the compiler to perform specific mathematical or logical operations. Operators in programming languages are taken from mathematics.
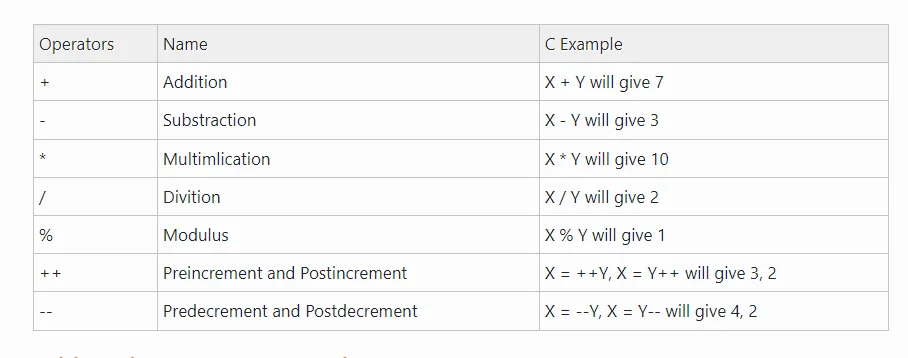
Relational operators
These operators are used to compare values and always result in boolean value (True or False). The following is a table of relational operators in C. Suppose you have two integer variables X, Y and having values 5, 2 respectively then
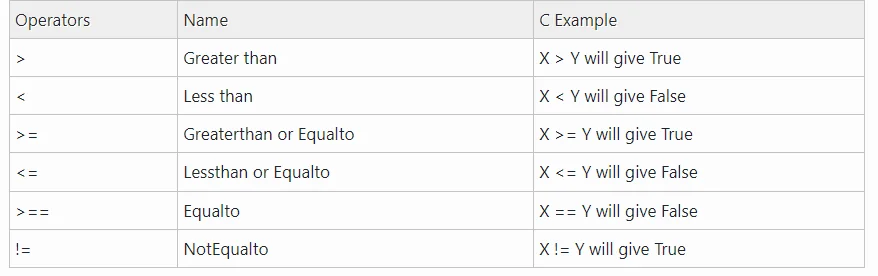
Logical Operators
These operators are used to compare values and always result in boolean value (True or False). The following is a table of logical operators in C. Suppose you have two boolean variables X, Y and having values True, False respectively then
These operators are used to perform logical operations on the given two variables.
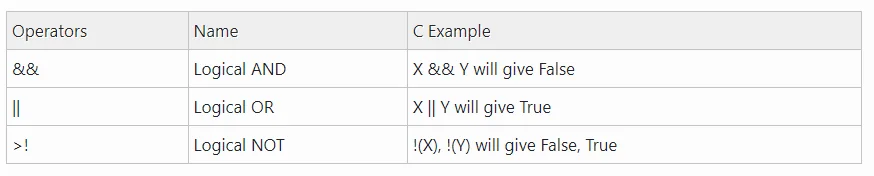
Assignment Operators
These operators are used to assign a new value to a variable, a property, an event, or an indexer element. The following is a table of assignments operators in C. Suppose you have two integer variables X, Y and having values 5, 2 then
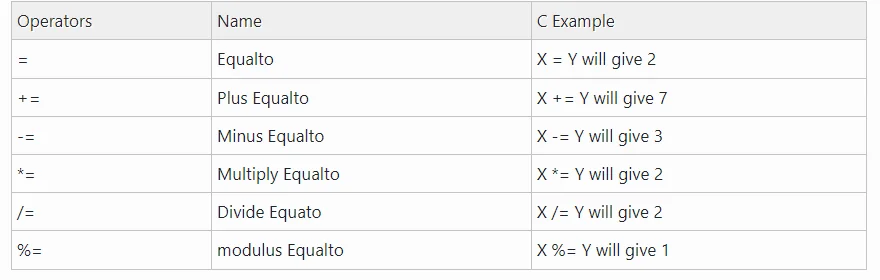
Bitwise Operators
These operators work on bits of a binary number and perform bit by bit operation. The following is a table of bitwise operators in C. Suppose you have two integer variables X, Y and having values 4, 5 respectively and theirs binary equivalent would be 100, 101 then
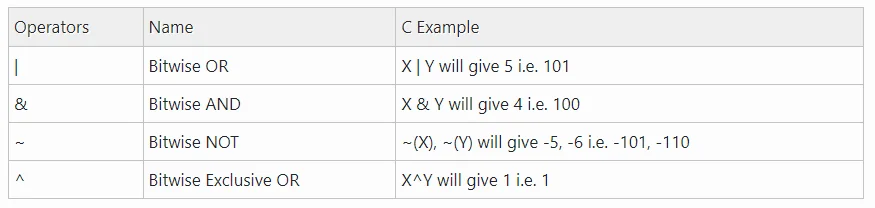
C Operators Precedence
Generally, arithmetic, logical, and relational operators are used while coding in C. The precedence for these operators in arithmetic is greater than logical and relational. Note that all the operators in arithmetic also follow a different order of precedence. Let’s check which operators hold the highest precedence.
Order of Precedence in Arithmetic Operators
The increment and decrement (+ + and – -) operators hold the highest precedence in arithmetic operators. After that next precedence is for the unary minus ( – ) operator; next, three operators, /, *, and %, have equal precedence. The lowest precedence is for the operators like addition ( + ) and subtraction ( – ). In case of equal priority, the compiler takes charge while evaluating them. Remember the C operator associativity rule, which is for all operators with the same precedence. Then the execution happens from left to right.
For example,
#include <stdio.h> int main() { int a = 15, b = 20, c = 32, result; result = a * b - ++c; printf("The result is: %d", result); return 0; }
Explanation: Here, in the given equation, first, “++” executes; hence the value of c will be 33. Next, “* “holds the highest precedence after “++”. Hence after the execution of “a * b,” the result will be 300. Then the execution of “-” happens and results in 267.
Order of Precedence in Relational/Logical Operators
The highest precedence in relational/logical operators is logical, not (!). After that, greater than (>), greater than equal to (>=), less than (<), and less than equal to (<=) operators hold equal precedence. Then “= =” and “ != ” operators hold the equal precedence. Next comes the logical And (&&) operator. In the end, logical OR (||) holds precedence. Even for relational and logical operators, the execution of those operators who hold the same precedence happens by the compiler.
For example,
10 > 1 + 8
Output: False
Explanation: Here, we know the arithmetic operator holds the highest precedence. Hence the execution of “1 + 8” happens first. It results in 9. Next, comparing the numbers happens: “10 > 9”. So the final result will be False as 10 is not greater than 9.
Misc Operators in C
The Misc operators or miscellaneous operators are conditional operators that include three operands. In these 3, the execution of the first operand happens first. Then the execution of the second operand, if it is non-zero, or a third operand executes to provide the necessary Output. Besides the operators discussed above, C programming language supports a few other special operators like sizeof and “?:”.
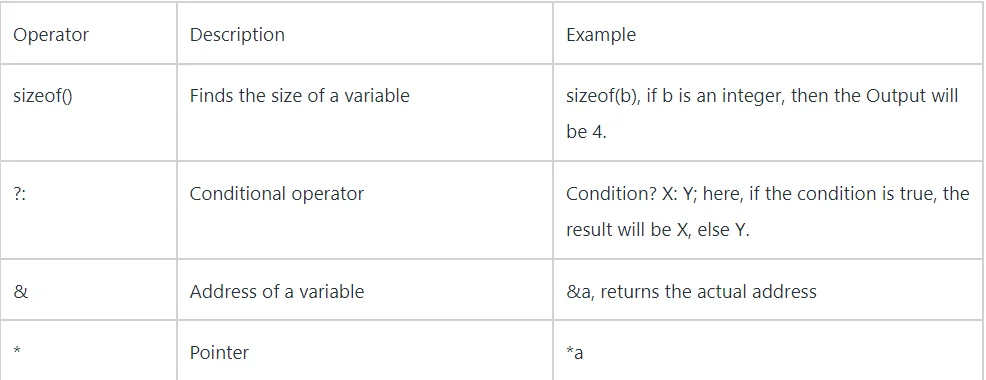
Time and Space Complexity
Time and space complexity are the terms concerning the execution of an algorithm. The Time complexity is the time taken to run the algorithm as a function of the input. Space complexity is the space or memory the algorithm takes as an input function. These two terms depend on many terms like processor, operating system, etc.
Final Thoughts
C operators are the symbols used to perform relational, mathematical, bitwise, or logical operations. C language includes a lot of operators to perform various tasks as necessary in the program. Different kinds of operators are arithmetic, logical, and relational. Learning C has a lot of benefits, After that, you can prepare for C language tutorial that can help you gain the job-ready skills you need to get started in the exciting field of programming.
0 Comments